Number to Thaana Converter for Rufiyaa
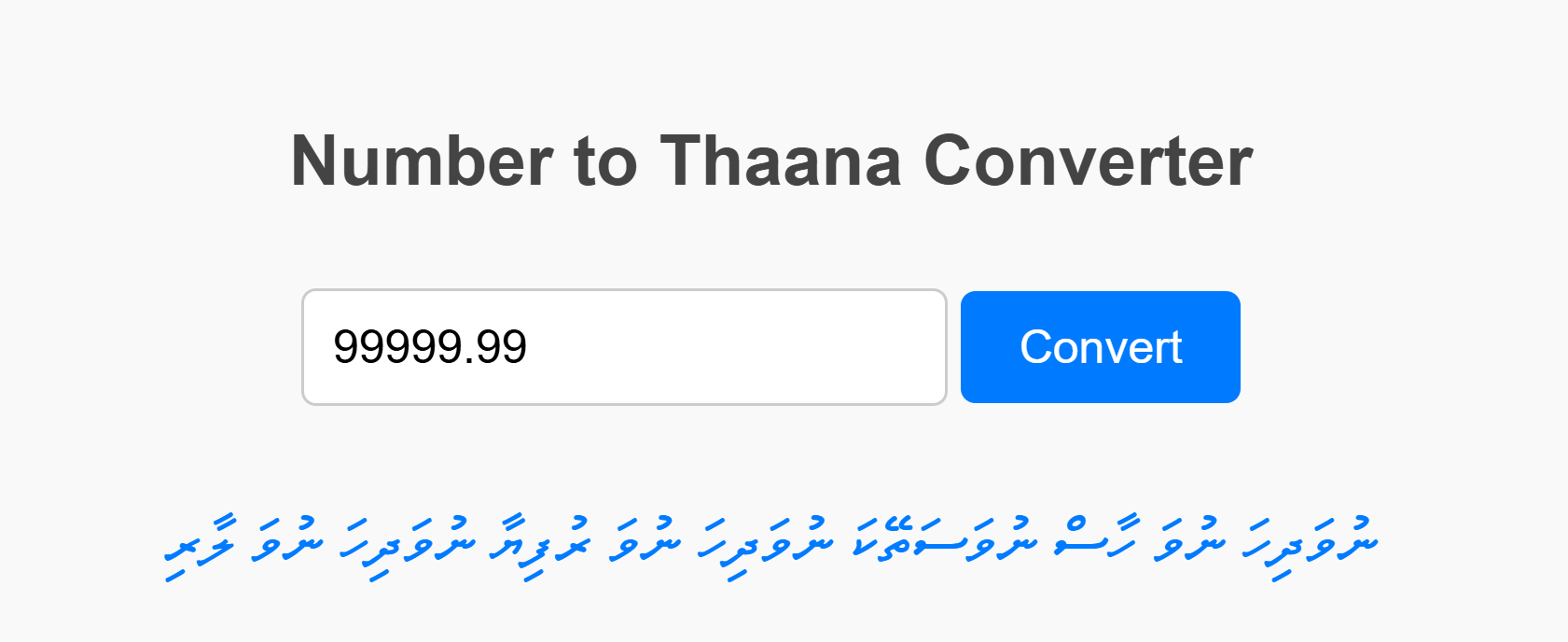
The Thaana script is used in the Maldives for the Dhivehi language, and converting numbers into Thaana, especially for Rufiyaa (ރުފިޔާ), is a unique and useful tool. In this guide, we'll show you how to create a fully functional "Number to Thaana Converter" app using JavaScript and HTML.
Features of the App
- Converts numbers into Thaana text.
- Handles decimal points to include laari (ލާރި).
- Supports numbers up to trillions with correct Thaana wordings.
Step-by-Step Implementation
1. Basic HTML Structure
We start with a clean HTML file for the UI. The layout includes:
- An input box to enter numbers.
- A button to trigger the conversion.
- A result area to display the converted Thaana text.
Here’s the HTML code:
<!DOCTYPE html>
{
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Number to Thaana Converter</title>
<style>
bodyfont-family
: Arial, sans-serif;text-align
: center;background-color: #f9f9f9
;color: #333
;padding: 20px
;
}h1
{font-size: 24px
;color: #444
;
}input[type="text"]
{padding: 10px
;font-size: 16px
;margin: 10px 0
;width: 200px
;border: 1px solid #ccc
;border-radius: 5px
;
}button
{padding: 10px 20px
;font-size: 16px
;color: #fff
;background-color: #007bff
;border
: none;border-radius: 5px
;cursor
: pointer;
}button:hover
{background-color: #0056b3
;
}#result
{margin-top: 20px
;font-size: 20px
;color: #007bff
;
}</style>
</head>
<body>
<h1>Number to Thaana Converter</h1>
<input type="text" id="numberInput" placeholder="Enter a number (e.g., 1.50)" />
<button onclick="convertToThaana()">Convert</button>
<div id="result"></div>
</body>
</html>
2. JavaScript Logic
a. Thaana Number Arrays
We define arrays for:
- Units (
ehbari
): Numbers 1-29. - Tens (
dhihabari
): Multiples of 10. - Place Values (
sunbari
): Thousand, million, etc.
javascript
const ehbari = ["", "އެއް", "ދެ", "ތިން", "ހަތަރު", "ފަސް", "ހަ", "ހަތް", "އަށް", "ނުވަ", "ދިހަ", "އެގާރަ", "ބާރަ", "ތޭރަ", "ސާދަ", "ފަނަރަ", "ސޯޅަ", "ސަތާރަ", "އަށާރަ", "ނަވާރަ", "ވިހި", "އެކާވީސް", "ބާވީސް", "ތޭވީސް", "ސައުވީސް", "ފަންސަވީސް", "ސައްބީސް", "ހަތާވީސް", "އަށާވީސް", "ނަވާވީސް"
];const dhihabari = ["ސުން", "ދިހަ", "ވިހި", "ތިރީސް", "ސާޅީސް", "ފަންސާސް", "ފަސްދޮޅަސް", "ހައްދިހަ", "އައްޑިހަ", "ނުވަދިހަ"
];const sunbari = ["", "ހާސް", "މިލިޔަން", "ބިލިޔަން", "ޓްރިލިޔަން"
];
b. Conversion Logic
We break the number into thousands, hundreds, and units, converting each part recursively.
javascript
function thousandSub(number
) { if (number <= 0 || number <= 29
) { return
ehbari[number]; } else if (number <= 99
) { return dhihabari[Math.floor(number / 10)] + (number % 10 !== 0 ? " " + ehbari[number % 10] : ""
); } else
{ const hundreds = Math.floor(number / 100
); const remainder = number % 100
; return ehbari[hundreds] + "ސަތޭކަ " + thousandSub
(remainder);
}
}function thousandUp(number
) { const
chunks = []; while (number > 0
) { chunks.push(number % 1000
); number = Math.floor(number / 1000
);
} return chunks.reverse().map((chunk, index) => thousandSub(chunk) + " " + sunbari[chunks.length - 1 - index]).join(" ").trim
();
}
c. Full Conversion
Combine the integer and decimal parts into Rufiyaa and Laari.
javascript
function convertToThaana(
) { const numberInput = document.getElementById("numberInput").value.trim
(); if (!numberInput || isNaN(parseFloat
(numberInput))) { document.getElementById("result").innerText = "Please enter a valid number!"
; return
;
} const [integerPart, decimalPart] = numberInput.split("."
); const number = parseInt(integerPart, 10
); const laari = decimalPart ? parseInt(decimalPart.padEnd(2, "0"), 10) : 0
; let result = thousandUp(number) + " ރުފިޔާ"
; if (laari > 0) result += " " + thousandSub(laari) + " ލާރި"
; document.getElementById("result").innerText
= result;
}
Try It Yourself
The full code is available above—copy, paste, and run it in your browser to test the "Number to Thaana Converter" for Rufiyaa. Let us know your thoughts! 😊