How to Built a Thaana Text Converter App
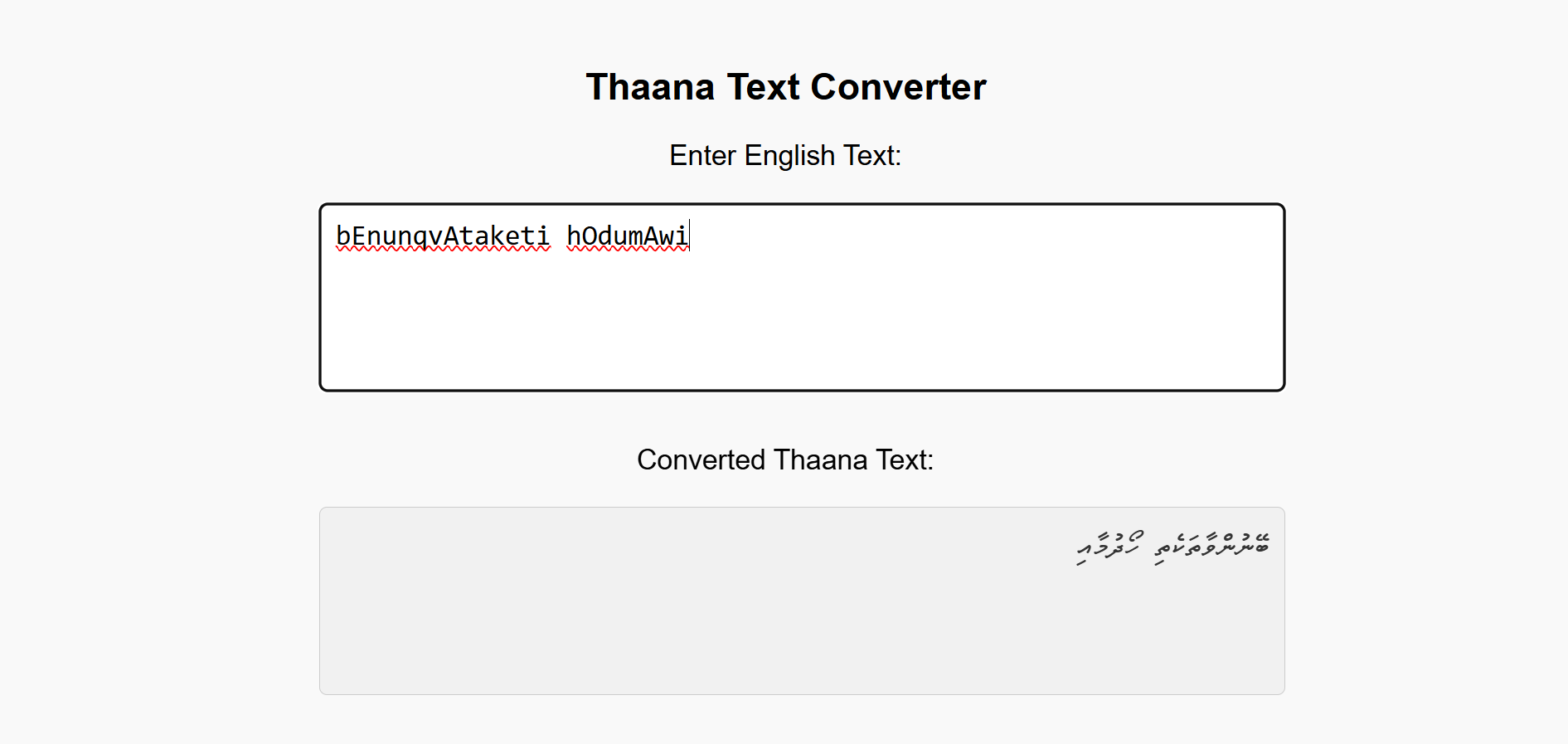
Recently, I embarked on a fun and challenging project: creating a Thaana text converter app. Thaana is the script used in the Dhivehi language, and I wanted to make it easier for people to type Thaana using English letters. In this blog post, I'll walk you through the process of building the app, explaining the code and design choices along the way.
Overview of the App
The app has a simple interface with two text areas:
- Input Field: Where users type English characters.
- Output Field: Displays the corresponding Thaana script in real-time.
Using JavaScript, the app converts English letters into Thaana script based on a predefined mapping.
Step-by-Step Guide to Building the App
1. Setting Up the HTML Structure
The HTML structure includes two text areas for input and output, wrapped in a simple container for styling.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Thaana Text Converter</title>
<style>
body {
font-family: Arial, sans-serif;
background-color: #f9f9f9;
padding: 20px;
}
.container {
max-width: 600px;
margin: 0 auto;
text-align: center;
}
textarea {
width: 100%;
height: 100px;
font-size: 18px;
padding: 10px;
margin: 10px 0;
border: 1px solid #ccc;
border-radius: 5px;
resize: none;
}
.output {
background-color: #f1f1f1;
color: #333;
direction: rtl;
text-align: right;
}
h1 {
font-size: 24px;
margin-bottom: 20px;
}
label {
font-size: 18px;
display: block;
margin-top: 20px;
margin-bottom: 10px;
}
</style>
</head>
<body>
<div class="container">
<h1>Thaana Text Converter</h1>
<label for="inputText">Enter English Text:</label>
<textarea id="inputText" placeholder="Type in English..."></textarea>
<label for="outputText">Converted Thaana Text:</label>
<textarea id="outputText" class="output" readonly></textarea>
</div>
</body>
</html>
Key Points:
- The
inputText
textarea accepts English input. - The
outputText
textarea displays the converted Thaana text. - The
direction: rtl;
style ensures that the Thaana text is displayed right-to-left.
2. Creating the Thaana Conversion Logic
The core functionality of the app is implemented in JavaScript. A mapping of English characters to Thaana characters is used to perform the conversion.
class ThaanaConverter {
constructor() {
this.mapping = {
q: "ް", w: "އ", e: "ެ", r: "ރ", t: "ތ", y: "ޔ", u: "ު", i: "ި", o: "ޮ", p: "ޕ",
a: "ަ", s: "ސ", d: "ދ", f: "ފ", g: "ގ", h: "ހ", j: "ޖ", k: "ކ", l: "ލ",
z: "ޒ", x: "×", c: "ޗ", v: "ވ", b: "ބ", n: "ނ", m: "މ",
Q: "ޤ", W: "ޢ", E: "ޭ", R: "ޜ", T: "ޓ", Y: "ޠ", U: "ޫ", I: "ީ", O: "ޯ", P: "÷",
A: "ާ", S: "ށ", D: "ޑ", F: "ﷲ", G: "ޣ", H: "ޙ", J: "ޛ", K: "ޚ", L: "ޅ",
Z: "ޡ", X: "ޘ", C: "ޝ", V: "ޥ", B: "ޞ", N: "ޏ", M: "ޟ",
",": "،", ";": "؛", "?": "؟", "<": ">", ">": "<", "[": "]", "]": "[",
"(": ")", ")": "(", "{": "}", "}": "{"
};
}
convert(text) {
let result = "";
for (const char of text) {
result += this.mapping[char] || char;
}
return result;
}
}
How It Works:
- The
ThaanaConverter
class defines amapping
object that maps English keys to Thaana characters. - The
convert
method loops through the input text and replaces each character with its Thaana equivalent using the mapping.
3. Connecting the Input and Output Fields
Next, I added event listeners to detect when users type in the input field. The text is converted to Thaana and displayed in the output field in real-time.
const converter = new ThaanaConverter();
const inputText = document.getElementById("inputText");
const outputText = document.getElementById("outputText");
inputText.addEventListener("input", () => {
const englishText = inputText.value;
const thaanaText = converter.convert(englishText);
outputText.value = thaanaText;
});
Key Points:
- The
input
event triggers every time the user types in the input field. - The
convert
method is called to translate the English input to Thaana. - The converted text is displayed in the
outputText
field.
Final Output
When users type English characters like tAna
, the app instantly converts it to ތާނަ
. This real-time feedback ensures an intuitive user experience.
Challenges Faced
- Character Mapping: Creating an accurate mapping of English characters to Thaana was a meticulous task.
- Right-to-Left Text Display: Ensuring the Thaana script displayed correctly in the
outputText
field required setting thedirection: rtl;
style.
Conclusion
This Thaana converter app is a simple yet powerful tool that makes it easy to type in Thaana using an English keyboard. By combining HTML, CSS, and JavaScript, I was able to create an intuitive interface and efficient conversion logic. Try building your own version or extending this app to add more features!